[文章作者:张宴 本文版本:v1.0 最后修改:2012.02.16 转载请注明原文链接:http://blog.zyan.cc/taobaoke_click_urls/]
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:
下载文件
申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。
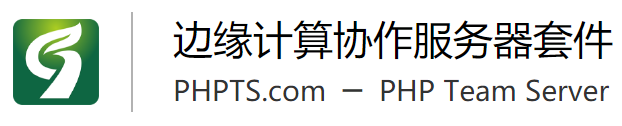
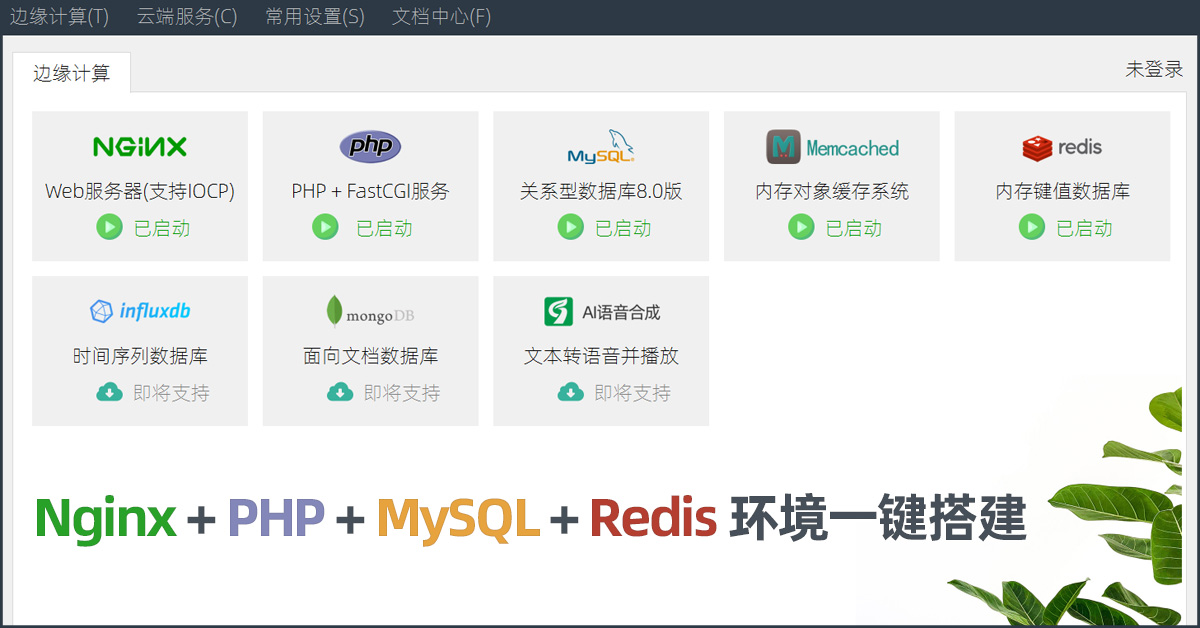
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
<?php
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:

申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。

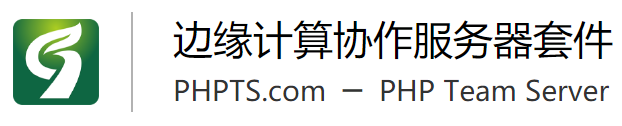
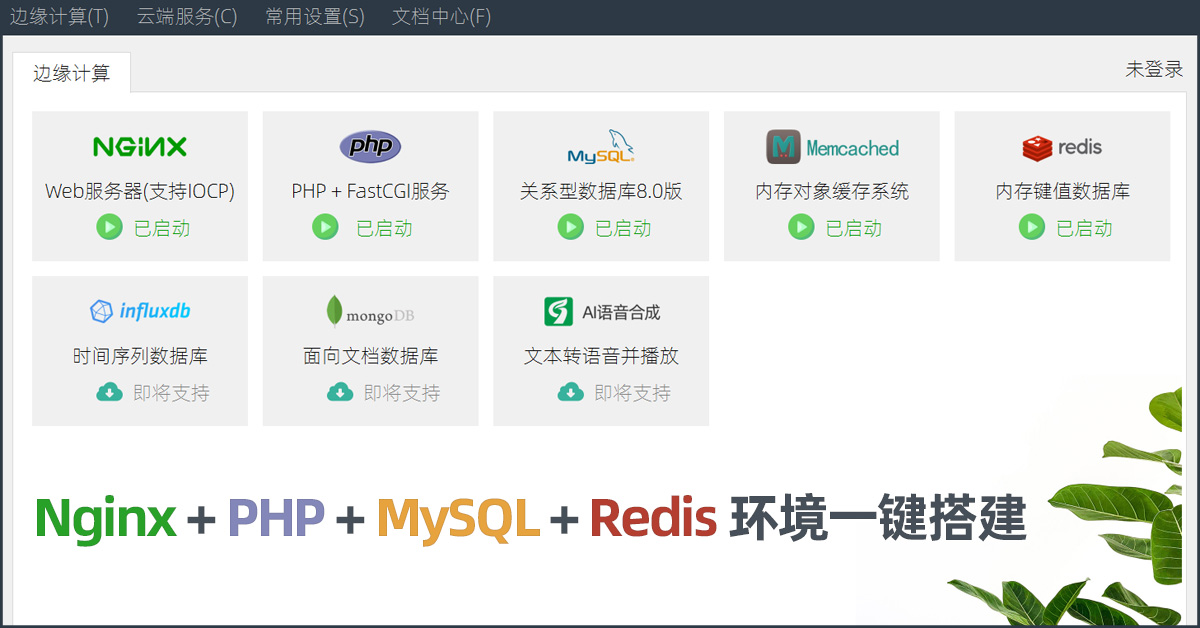
สล็อต pg
2023-7-26 18:34
Can you สล็อต pg เว็บตรง ไม่ผ่านเอเย่นต์ ไม่มี ขั้น ต่ํา imagine the excitement of discovering new and exclusive slot titles regularly added to the direct web collection?
https://tga168.vip
2023-7-26 18:34
Can you https://tga168.vip/ envision the excitement of competing for leaderboard positions and earning prestigious rewards on the direct web slots?
June11
2023-8-11 17:12
슬롯사이트카지노사이트슬롯사이트
June11
2023-8-11 17:12
카지노사이트슬롯사이트카지노사이트
Rolando Bass

2023-8-24 15:22
download and install spotify premium mod apk última versión to enjoy the songs of today's top singers in the world
lucabet168


2023-8-25 02:02
"เว็บเดิมพันสล็อตออนไลน์ขวัญใจคอเกมสล็อต บาคาร่า มาแรงอันดับ 1ในตอนนี้ ในเรื่องคุณภาพ การบริการ และมาตรฐานรองรับระดับสากล ร่วมสัมผัสประสบการณ์เดิมพัน <a href="https://lucabet168plus.bet/" rel="nofollow ugc">lucabet168</a> ใหม่ล่าสุด 2022 แตกหนักทุกเกม ทำกำไรได้ไม่อั้น และโปรโมชั่นที่ดีที่สุดในตอนนี้ นักเดิมพันที่กำลังมองหาช่องทางสร้างรายได้เสริมและความเพลิดเพลินอย่างไม่มีที่สิ้นสุด ที่นี่เรามีเกมสล็อต คาสิโน หวย <a href="https://lucabet168plus.bet/" rel="nofollow ugc">สล็อตเว็บตรง</a> ให้คุณเลือกเล่นไม่อั้นนับ 1,000+ เกม และรวมคาสิโนไว้แล้วที่นี่ เพียงเข้ามาสมัครสมาชิกก็ร่วมสนุกกับเกม PG POCKET GAMES SLOT ฝากถอนไม่มีขั้นต่ําด้วยระบบออโต้ ที่สุดแห่งความทันสมัย เชื่อถือได้ และรวดเร็วที่สุด คีย์ของเราพร้อม lucabet168 สล็อตเว็บตรง
lava95


2023-8-25 06:15
"เว็บเดิมพันสล็อตออนไลน์ขวัญใจคอเกมสล็อต มาแรงอันดับ 1ในตอนนี้ ในเรื่องคุณภาพ การบริการ และมาตรฐานรองรับระดับสากล ร่วมสัมผัสประสบการณ์เดิมพัน <a href="https://lava95.com/" rel="nofollow ugc">pgslot</a> ใหม่ล่าสุด 2022 แตกหนักทุกเกม ทำกำไรได้ไม่อั้น และโปรโมชั่นที่ดีที่สุดในตอนนี้ นักเดิมพันที่กำลังมองหาช่องทางสร้างรายได้เสริมและความเพลิดเพลินอย่างไม่มีที่สิ้นสุด ที่นี่เรามีเกมสล็อต คาสิโน หวย<a href="https://lava95.com/" rel="nofollow ugc">สล็อตเว็บตรง</a> ให้คุณเลือกเล่นไม่อั้นนับ 1,000+ เกม และรวมคาสิโนไว้แล้วที่นี่ เพียงเข้ามาสมัครสมาชิกก็ร่วมสนุกกับเกม PG POCKET GAMES SLOT ฝากถอนไม่มีขั้นต่ําด้วยระบบออโต้ ที่สุดแห่งความทันสมัย เชื่อถือได้ และรวดเร็วที่สุด คีย์ของเราพร้อม pgslot สล็อตเว็บตรง
nolahitchcock
2023-9-6 11:41
install now potify premium última versión to get the latest songs we just updated
LucyGray
2023-10-28 11:45
Congratulations on your valuable contribution to the community through this article. Download game apk with diverse themes that I like the most.
katmovies

2023-10-30 01:25
This is my first visit to your web journal! I really enjoyed your blog Thanks for sharing.katmovies
aaareplicatrade


2023-11-7 20:46
I wanted to thank you for this great read!! I definitely enjoying every little bit of it I have you bookmarked to check out new stuff you post. replica clothes
beverlysilva
2023-11-24 15:14
Great. Experience the MOBILE APK to have the most accurate views
Foxi Apk

2024-1-4 20:02
Nice blog buddy. I like your all post. Foxi Apk Download
Lucky cola
2024-2-7 16:38
Are you ready to become a gaming legend? Lucky cola
Hattie Mendoza

2024-3-5 16:38
Great. Experience the latest features of Spotify Premium APK that will not disappoint you
Jimmi jack

2024-4-4 01:55
Thanks for sharing. its absolutely worth it. However, if you want to enjoy all the features for free, I recommend you to download the latest version of Spotify Premium Apk. Thank you for your recommendations as well. here you can also find one of the most famous and great singers. Thanks to the team Spotify Premium Apk
Spoti Geek


2024-4-7 18:26
Thanks for sharing! With Spotify Premium APK, you can listen to lots of great music by famous singers. It's a special version of Spotify where you get even more songs and features for an awesome music experience of.Spotify Premium APK
Spoti Geek


2024-4-7 18:27
Thanks for sharing! With Spotify Premium APK, you can listen to lots of great music by famous singers. It's a special version of Spotify where you get even more songs and features for an awesome music experience of.Spotify Premium APK
Spoti Geek


2024-4-7 18:29
Thanks for sharing! With Spotify Premium APK, you can listen to lots of great music by famous singers. It's a special version of Spotify where you get even more songs and features for an awesome music experience of Spotify Premium Apk
cricapkmod


2024-8-2 03:07
Dive into the world for apk mods click here
分页: 11/12
6 7 8 9 10 11 12



