[文章作者:张宴 本文版本:v1.0 最后修改:2012.02.16 转载请注明原文链接:http://blog.zyan.cc/taobaoke_click_urls/]
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:
下载文件
申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。
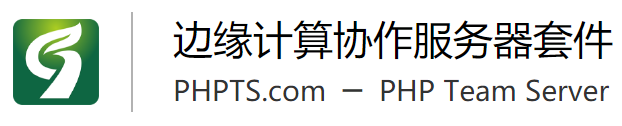
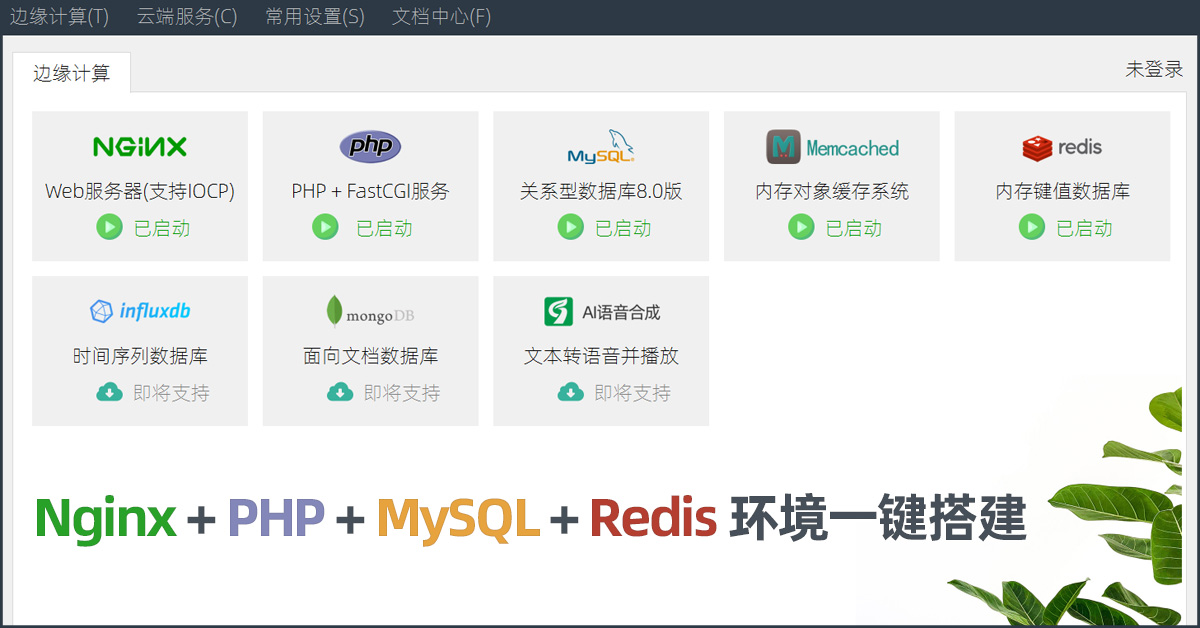
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
<?php
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:

申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。

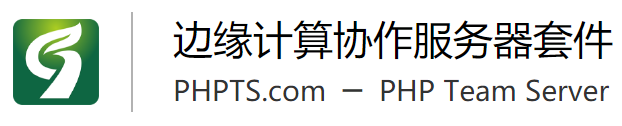
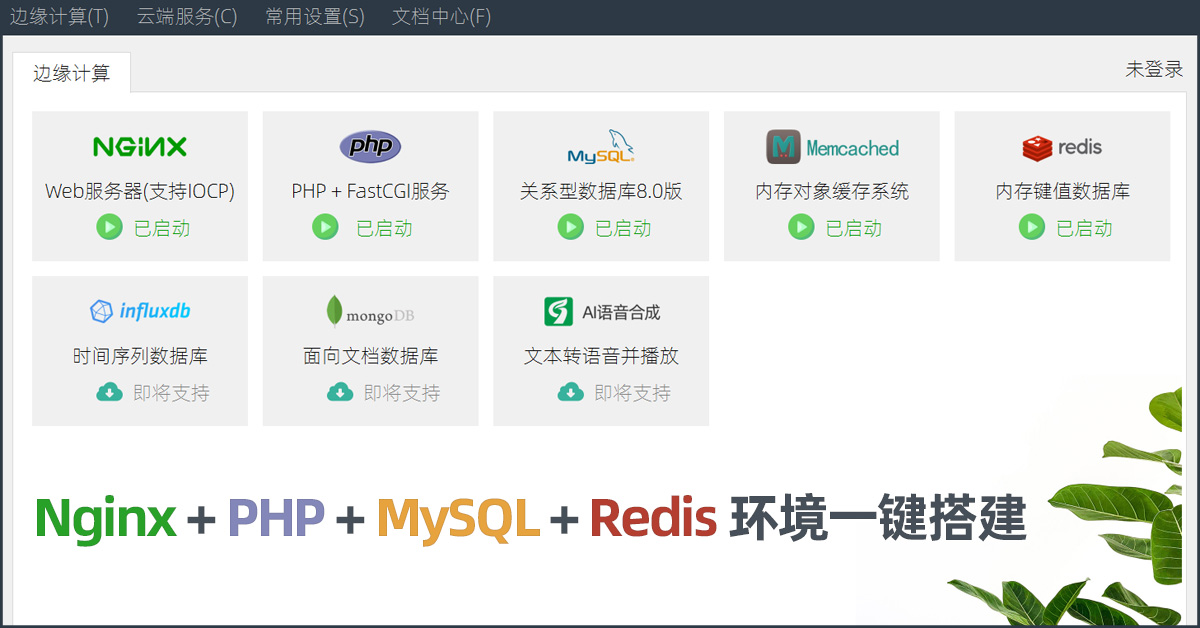
cattle nets", and click "online voting" found that, the original vote is paid, 1 yuan 1 ticket.
"If you have to vote online, by pay treasure, net silver or cell phone pays fee." HeXianSheng cheap designer clothes
said, let people will choose the doctors like this is a good thing, but if will pay it is stale, "so, mean who's money, who can election?"
【 operation 】 can buy tickets into batch quantity
discount designer handbags
According to HeXianSheng provide web site, reporter found "purple cattle nets", "popular activities" column in the article 1 is: ningxiang county "open the door the medical" advanced unit and "top ten doctors, top ten nurses, top ten technicians" selection activities to officiall
例如 ID:111111111 衣服类
ID:111111112 裤子类
这种的分类
<a href="http://www.officialbvbags.com/" title="Bottega Veneta Bags"><h1>Bottega Veneta Bags</h1></a>
<a href="http://www.officialbvbags.com/" title="Bottega Veneta Handbags"><h1>Bottega Veneta Handbags</h1></a>