[文章作者:张宴 本文版本:v1.0 最后修改:2012.02.16 转载请注明原文链接:http://blog.zyan.cc/taobaoke_click_urls/]
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:
下载文件
申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。
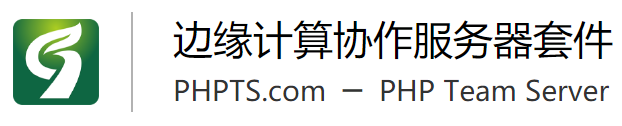
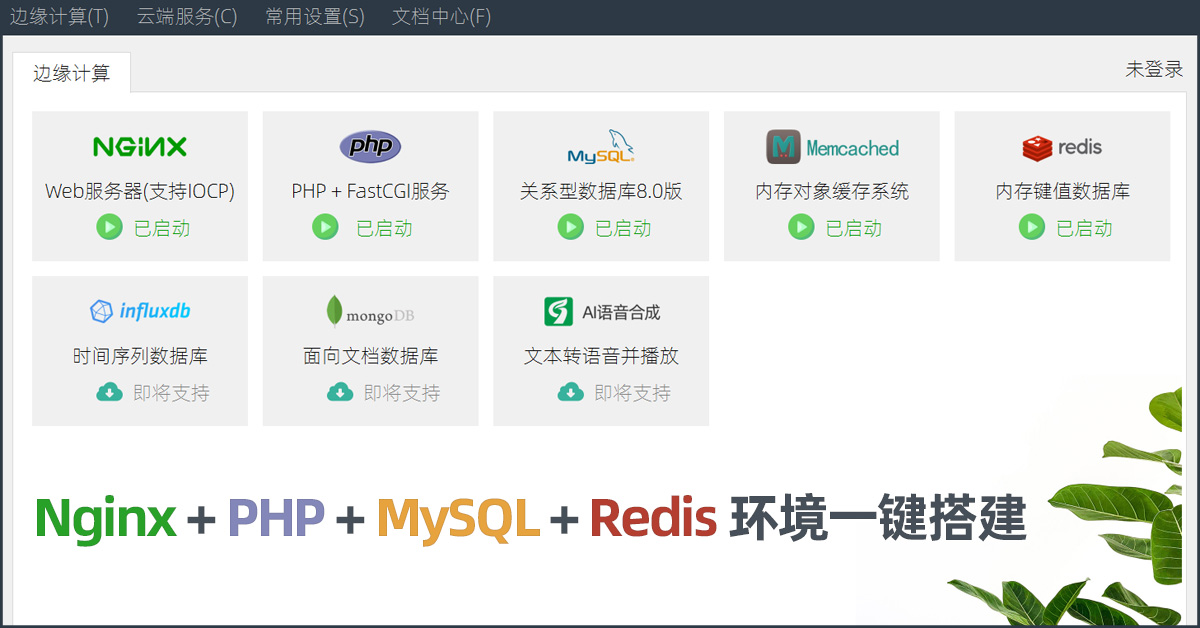
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
<?php
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:

申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。

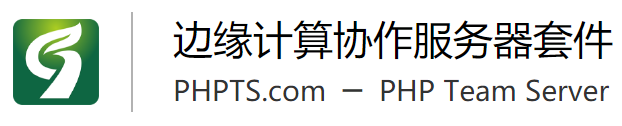
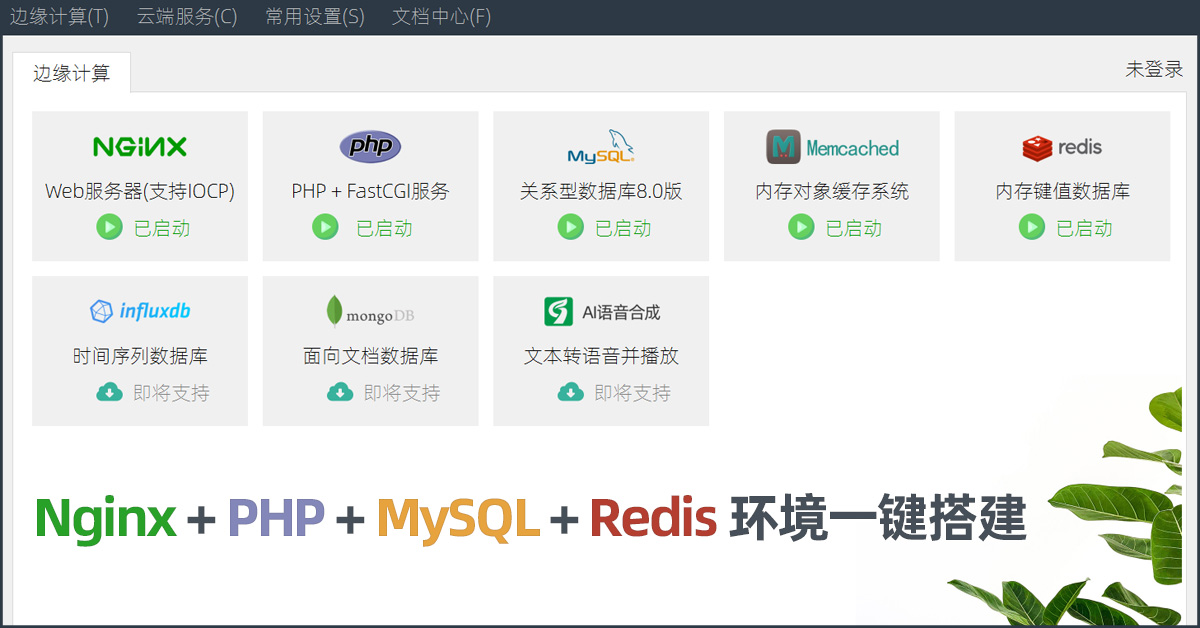
the one


2021-12-14 18:38
cakes swaziland eswatinibakeries in swaziland
AMKHG

2021-12-15 00:53
LikeMyChoice is a boutique online brand that aims to offer a unique, vintage selection of fashion and timeless classics. At LikeMyChoice, you can find all trendy products at most affordable price with Express Shipping options across the world. Like My Choice
AMKM

2021-12-16 01:57
You have beaten yourself this time, and I appreciate you and hopping for some more informative posts in future. Thank you for sharing great information to us. gclub
AMK

2021-12-17 13:49
You have a real ability for writing unique content. I like how you think and the way you represent your views in this article. I agree with your way of thinking. Thank you for sharing. effects of stress on the brain
seo

2021-12-18 03:48
Thank you for taking the time to publish this information very useful! Royal Online V2
anveshj


2021-12-20 18:31
Thanks for the best share and i loved it,https://19216811.support/
Akash Sinha

2021-12-27 13:51
Pretty good post. I have just stumbled upon your blog and enjoyed reading your blog posts very much. I am looking for new posts to get more precious info. Big thanks for the useful info. moviesflix
Hibbah

2022-1-2 14:27
Thank you for another great article. Where else could anyone get that kind of information in such a perfect way of writing? I have a presentation next week, and I am on the look for such information. Dankwoods blunt
seo

2022-1-8 00:31
Your web log isn’t only useful but it is additionally really creative too. hifu
JMKO

2022-1-14 18:10
I think this is one of the most significant information for me. And i’m glad reading your article. But should remark on some general things, The web site style is perfect, the articles is really great : D. Good job, cheers dankwoods
Daily news
2022-2-3 19:07
Thanks for the PHP function to generate Taobao guest links in batches.
Daily news
2022-2-3 19:09
Thanks for the PHP function to generate Taobao guest links in batches. 9xflix
Hibbah

2022-2-7 19:34
Thanks, that was a really cool read! what energy drink has the most caffeine
Hibbah

2022-2-9 10:27
Public debt relief has been around for further than a decade and has helped a lot of debtors to pay off their debts in lower quantities than they owe. You don't have anything to deposit before the process starts with the debt operation plan they give you. Credit Card Debt Relief
Hibbah

2022-2-14 19:25
I havent any word to appreciate this post.....Really i am impressed from this post....the person who create this post it was a great human..thanks for shared this with us. Find out more about the best stationery supplier
brucesmith66

2022-2-15 13:41
Thanks for the motivating and insightful piece of composing. It is Very thorough and informative description. It's not easy to discover such useful idears in the net. hp 204a should remain in the information on the make-up, and also not simply on the blog site! We anticipate to finding out more from you.
AMKO

2022-2-15 14:15
Cappuccino MCT is a weight reduction supporting espresso. The Bulletproof espresso gets its name from the solid fat and different fixings that assist you with shedding pounds, control your hunger, and hone your psyche. Is Cappuccino MCT Good For Weight Loss?
seo

2022-2-16 06:24
When you use a genuine service, you will be able to provide instructions, share materials and choose the formatting style. 918kiss
seo

2022-2-16 06:25
Great job for publishing such a beneficial web site. Your web log isn’t only useful but it is additionally really creative too. sagame66
seo

2022-2-16 06:26
This is such a great resource that you are providing and you give it away for free. I love seeing blog that understand the value. Im glad to have found this post as its such an interesting one! I am always on the lookout for quality posts and articles so i suppose im lucky to have found this! I hope you will be adding more in the future... 918kiss
分页: 6/12
1 2 3 4 5 6 7 8 9 10



