[文章作者:张宴 本文版本:v1.0 最后修改:2012.02.16 转载请注明原文链接:http://blog.zyan.cc/taobaoke_click_urls/]
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:
下载文件
申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。
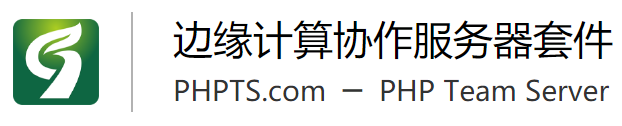
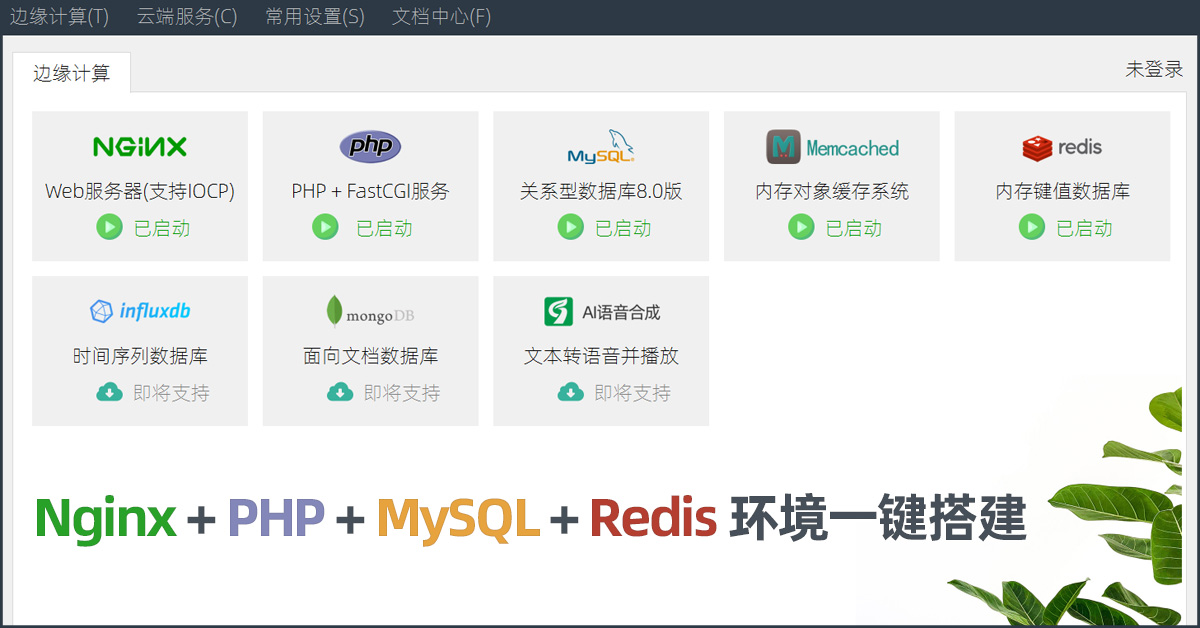
根据淘宝商品 num_iid 批量生成淘宝客(什么是淘宝客?)链接的 PHP 文件内容如下。
淘宝 API 有调用次数限制,一次 API 调用,可以最大返回40个商品的淘宝客链接,因此,在本函数内,如果需要批量生成的淘宝商品 num_iid 数大于40,将按照40个一次,分多次调用。如果调用淘宝 API 查询过的商品 num_iid,不管其是否有淘宝客链接(有些商品没有淘宝客推广链接),都将利用 Memcached 缓存起来,下次直接查缓存,不会重复调用淘宝 API。
<?php
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
require_once(dirname(__FILE__).'/TopSdk.php'); //引用淘宝开放平台 API SDK
function object2Array($d)
{
if (is_object($d))
{
$d = get_object_vars($d);
}
if (is_array($d))
{
return array_map(__FUNCTION__, $d);
}
else
{
return $d;
}
}
/*********************************************
* 函数名:get_taobaoke_link ($num_iids)
* 函数用途:通过淘宝商品 num_iids 获取其对应的淘宝客手机版链接
* 创建时间:2012-02-14
* 创建人:张宴 net@zyan.cc
* 参数说明:
* $num_iids 淘宝商品ID(支持多个商品)数组,示例如下:
* $num_iids[] = "13583512568";
* $num_iids[] = "10809380078";
* $num_iids[] = "10809380079";
* 返回值:
* 下标为淘宝商品 num_iid ,值为淘宝客链接 click_url 的二维数组。如果无淘宝客链接,click_url 为空字符串,示例如下:
* array(3) {
* ["13583512568"]=>
* string(191) "http://auction1.wap.taobao.com/auction/item_detail-0db2-13583512568.jhtml?tks=jUTwPLMDtUUNEZhqfEuTZqkZhGw1LA7%2BzCJBXCj27NpurHxjZN70Amg0DVaFU61pfnHwW%2FI4MZGm%0Awgb69kbb1NL8uwtu%2BDnyAunBCVDP"
* ["10809380078"]=>
* string(187) "http://auction1.wap.taobao.com/auction/item_detail-0db2-10809380078.jhtml?tks=jUTwPLMDtUUNEGWhOOgVVuX%2BJKYt7fesyuZjEe7hvmpTJxYDfK8i1Wpvfl7lwI7nzD9W8M352v6E%0AyuUtsKun81AGltKzJWCYPiVDiOeC"
* ["10809380079"]=>
* string(0) ""
* }
*********************************************/
function get_taobaoke_link ($num_iids) {
$memcache = new Memcache;
$memcache->connect('127.0.0.1', 11911); //Memcached 缓存服务器地址
$click_urls = $memcache->get($num_iids);
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$tbapi_num_iids_arr[] = $num_iid;
}
}
if (!empty($tbapi_num_iids_arr)) {
$numbers = count($tbapi_num_iids_arr);
$numbers_max = 40; //淘宝 API 限制最大返回40条记录
if ($numbers > 0) {
$numbers_times = ceil($numbers / $numbers_max); //第一层循环的循环次数
$numbers_start = 0;
$numbers_end = $numbers_max;
for ($numbers_i = 1; $numbers_i <= $numbers_times; $numbers_i++) {
for ($numbers_j = $numbers_start; $numbers_j < $numbers_end; $numbers_j++) {
if ($numbers_j >= $numbers) {
break;
}
$tbapi_num_iids_arr_sp[] = $tbapi_num_iids_arr[$numbers_j];
}
$numbers_start = $numbers_start + $numbers_max;
$numbers_end = $numbers_end + $numbers_max;
$tbapi_num_iids = implode(",", $tbapi_num_iids_arr_sp);
$c = new TopClient;
$c->appkey = 12498835; //淘宝开放平台 API 接口 App Key
$c->secretKey = "745db5f8e316f9f1aa8310a7568d6566"; //淘宝开放平台 API 接口 App Secret
$c->format = "json";
$req = new TaobaokeItemsConvertRequest;
$req->setFields("num_iid,click_url");
$req->setNumIids($tbapi_num_iids);
$req->setPid(29509662); //淘宝联盟(阿里妈妈)PID
$req->setIsMobile("true"); //如果要生成手机页面的淘宝客链接,选择 true;网页版选择 false
$resp = $c->execute($req);
$res = object2Array($resp);
if (isset($res["taobaoke_items"]["taobaoke_item"])) {
$links = $res["taobaoke_items"]["taobaoke_item"];
foreach ($links as $value) {
$memcache->set($value["num_iid"], $value["click_url"], MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$value["num_iid"]] = $value["click_url"];
}
}
unset($tbapi_num_iids_arr_sp);
unset($tbapi_num_iids);
unset($resp);
unset($res);
unset($links);
unset($value);
}
}
}
foreach ($num_iids AS $num_iid) {
if (!isset($click_urls[$num_iid])) {
$memcache->set($num_iid, "", MEMCACHE_COMPRESSED, 0);
$click_urls[(string)$num_iid] = "";
}
}
$memcache->close();
return $click_urls;
}
//演示
$num_iids[] = "13583512568";
$num_iids[] = "10809380078";
$num_iids[] = "10809380079";
$click_urls = get_taobaoke_link ($num_iids);
var_dump($click_urls);
?>
淘宝开放平台(http://open.taobao.com/) PHP SDK 下载:

申请淘宝 API 的 App Key 和 App Secret ,可以到 http://my.open.taobao.com/ 进行。

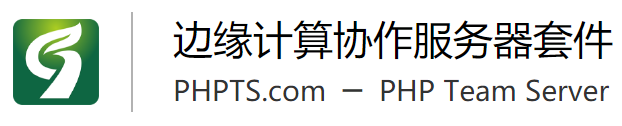
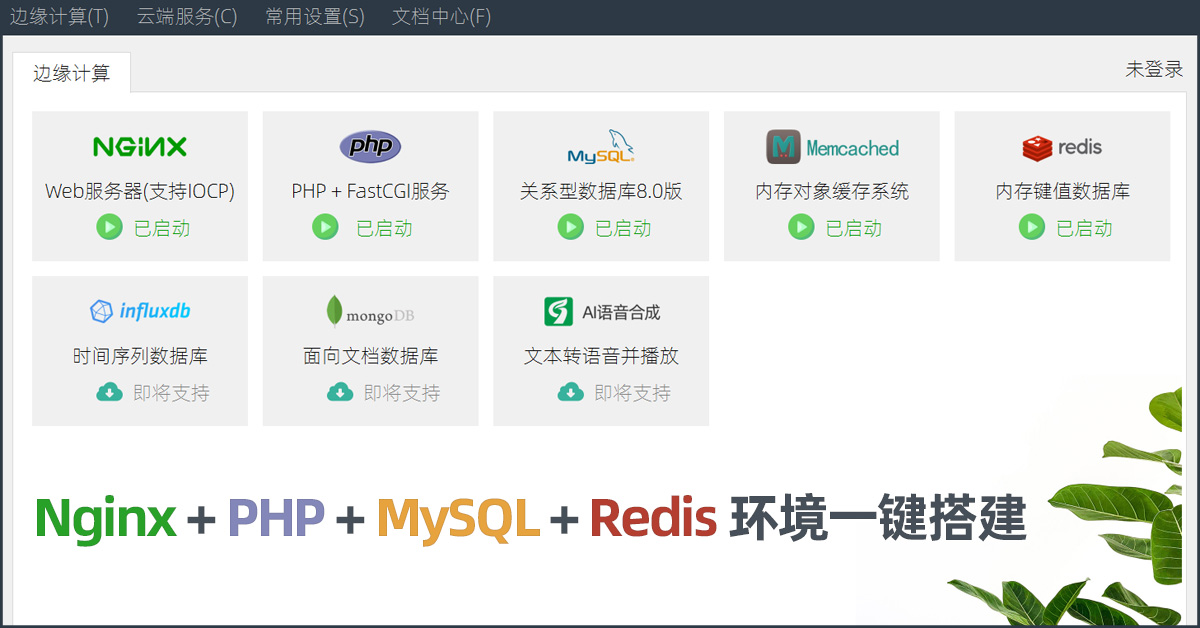
Daily news

2022-2-17 15:29
Cool stuff, Thank you very much and will look for more postings from you. 9xflix
Hibbah

2022-2-17 19:01
After the normal was taken, the supposed federal retirement aide factor was embedded . So, the more limited the commitment time and the age of the individual, the lower the advantage sum would be. Aposentadoria por tempo de contribuição antes da reforma
Hibbah

2022-2-20 14:56
I visit your blog regularly and recommend it to all of those who wanted to enhance their knowledge with ease. The style of writing is excellent and also the content is top-notch. Thanks for that shrewdness you provide the readers! walikota siantar
Hibbah

2022-2-21 11:08
ادوات صحية جمعية فنحنو لدينا افضل فنيين متخصصين في اعمال السباكة وتركيب الادوات الصحيه وتسليك المجاري سواء انسداد في حمامات او مطابخ – تركيب مضخات مياه – تركيب سخانات مركزيه وعادي – تركيب فلاتر مياه الشرب – تركيب شاور بوكس وجاكوزي – لدينا خبرات سابقه ودعم فني عاليه. ادوات صحية جميعه
HAY Day Mod Apk


2022-2-22 20:15
Besides from all action and fighting if one needs some peace and pure feelings of simple as well as charming life of villagers specially then, HAY Day Mod Apk game is surely for you. This is a farming game where player has to create its very own personalized farm.
สล็อตเว็บใหญ่ PG


2022-2-23 14:38
สล็อตเว็บใหญ่ PG currently has online slot games. Comes in a new format with 3D images as slots online, direct websites and leading game camps such as PG SLOT JOKER GAME, new member registration, 100% bonus and many more slots
pg 168


2022-2-23 14:39
pg 168 currently has online slot games. Comes in a new format with 3D images as slots online, direct websites and leading game camps such as PG SLOT JOKER GAME, new member registration, 100% bonus and many more slots
pg slot เว็บใหม่


2022-2-23 14:39
pg slot เว็บใหม่ online slot games The most popular of the year 2021, joker game, deposit-withdraw, no minimum, there is a popular game like roma slot, apply for a joker game today, get a 100% bonus if you ask about the hot online gambling game overtaking the curve.
สล็อต


2022-2-23 14:39
สล็อต online slot games The most popular of the year 2021, joker game, deposit-withdraw, no minimum, there is a popular game like roma slot, apply for a joker game today, get a 100% bonus if you ask about the hot online gambling game overtaking the curve.
Hibbah

2022-2-25 01:02
Hi there! Nice material, do keep me posted when you post something like this again! I will visit this blog leaps and bounds for more quality posts like it. Thanks... youlikebet
seoo

2022-3-2 23:26
I haven’t any word to appreciate this post.....Really i am impressed from this post....the person who create this post it was a great human..thanks for shared this with us. list of hospitals in the philippines
Sheri Warner


2022-3-4 10:34
Thanks for the useful information you share, download free ringtones with a lot of trending music genres right away klingeltöne kostenlos
seoo

2022-3-5 23:11
I learn some new stuff from it too, thanks for sharing your information. 656win
seoo

2022-3-5 23:17
I am impressed. I don't think Ive met anyone who knows as much about this subject as you do. You are truly well informed and very intelligent. You wrote something that people could understand and made the subject intriguing for everyone. Really, great blog you have got here. slot168.com
seo

2022-3-16 00:36
This is my first time visit here. From the tons of comments on your articles,I guess I am not only one having all the enjoyment right here! fb video downloader
seo

2022-3-21 03:47
I have read all the comments and suggestions posted by the visitors for this article are very fine,We will wait for your next article so only.Thanks! America's next mass migration
seoo

2022-3-26 03:39
You make so many great points here that I read your article a couple of times. Your views are in accordance with my own for the most part. This is great content for your readers. magazeeno.com
seo

2022-3-28 04:53
I would like to thank you for the efforts you have made in writing this article. I am hoping the same best work from you in the future as well. Thanks... เกม เสือ มังกร
Mayme Rome

2022-3-31 18:29
Thanks for sharing. You can listen to music on descargar spotify premium gratis for free and moreover there are many great songs from famous singers around the world.
PGGAMESLOT


2022-4-1 00:22
pg The latest with a game system designed for direct pg slot players because the game format is very advanced, can play pg slot auto via ios and android systems, supports playing pg slots via mobile Deposit-withdraw automatically in just 8 seconds
分页: 7/12
2 3 4 5 6 7 8 9 10 11



